Django - Adding Hot Reload (with sample)
Learn how to improve your development speed by adding the Hot Reload feature for templates and statics in a Django-based project.
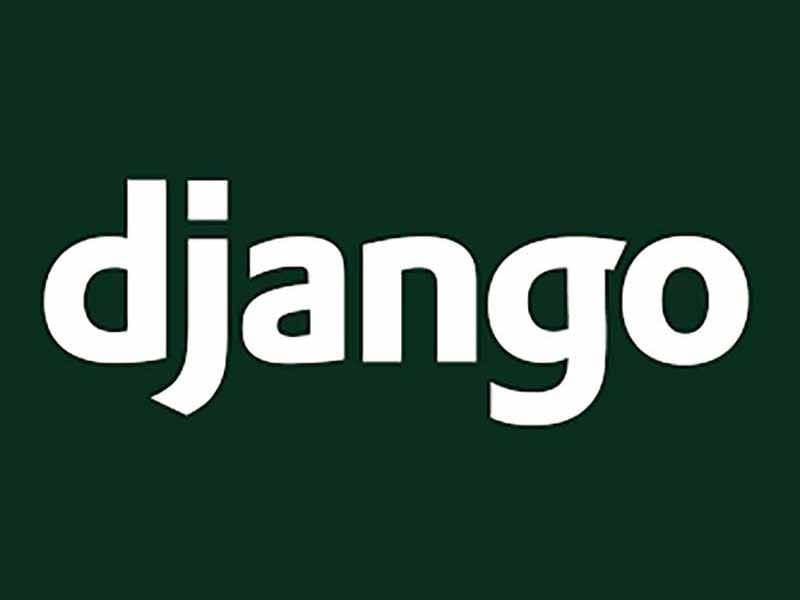
Hello Coders! Implementing hot reload for templates and static files in Django can greatly improve your development workflow. You can take a few different approaches to achieve hot reload in Django, and this article presents the most simple ones. To make this article more useful, in the end, an open-source sample is provided. Thanks for reading!
#1 - Watchgod Utility
One popular method is to use the django-extensions
package along with watchgod
for auto-reloading.
$ pip install django-extensions
Then, you can add django_extensions
to your INSTALLED_APPS
in your Django settings file (settings.py
).
For hot reloading of templates, you can use the runserver_plus
command provided by django-extensions
, which automatically reloads templates when they change.
$ python manage.py runserver_plus
For static files, you can use watchmedo
(a part of watchgod
) to monitor changes and trigger reloads:
$ watchmedo shell-command --patterns="*.css;*.js;*.html" --command="python manage.py collectstatic --noinput" --recursive static
This command watches for changes in your static files directory and triggers collectstatic
whenever a change is detected. Combined with Django's built-in development server, this provides a pretty seamless hot reload experience for both templates and static files.
#2 - Django-Browser-Reload
This open-source library can be integrated with any Django project with only a few lines of code as stated on the official PyPi page:
Install the library using PIP or any other package manager
$ pip install django-browser-reload
Make sure you have "django.contrib.staticfiles" in your INSTALLED_APPS section. Besides this, a new application should be added:
INSTALLED_APPS = [
...,
"django_browser_reload", # <-- NEW
...,
]
The next step is to update the project routing rules to include the library URLs:
from django.urls import include, path
urlpatterns = [
...,
path("__reload__/", include("django_browser_reload.urls")),
]
Updating the middleware is the last configuration step required for this integration.
MIDDLEWARE = [
# ...
"django_browser_reload.middleware.BrowserReloadMiddleware",
# ...
]
The middleware automatically inserts the required script tag on HTML responses before </body> when DEBUG is True.
If all the above steps were followed, our Django project should support the HOT reload feature, and all the changes we made to the templates and static files are automatically synced with the browser.
Hot Reload Sample
For those in a rush for a sample, Rocket Django, an open-source Tailwind-based starter provides already the integration for Django-Browser-Reload Library:
- dependency added in the requirements.txt file
- settings file [ INSTALLED_APPS and MIDDLEWARE sections ]
- routing [ "django_browser_reload.urls" included ]
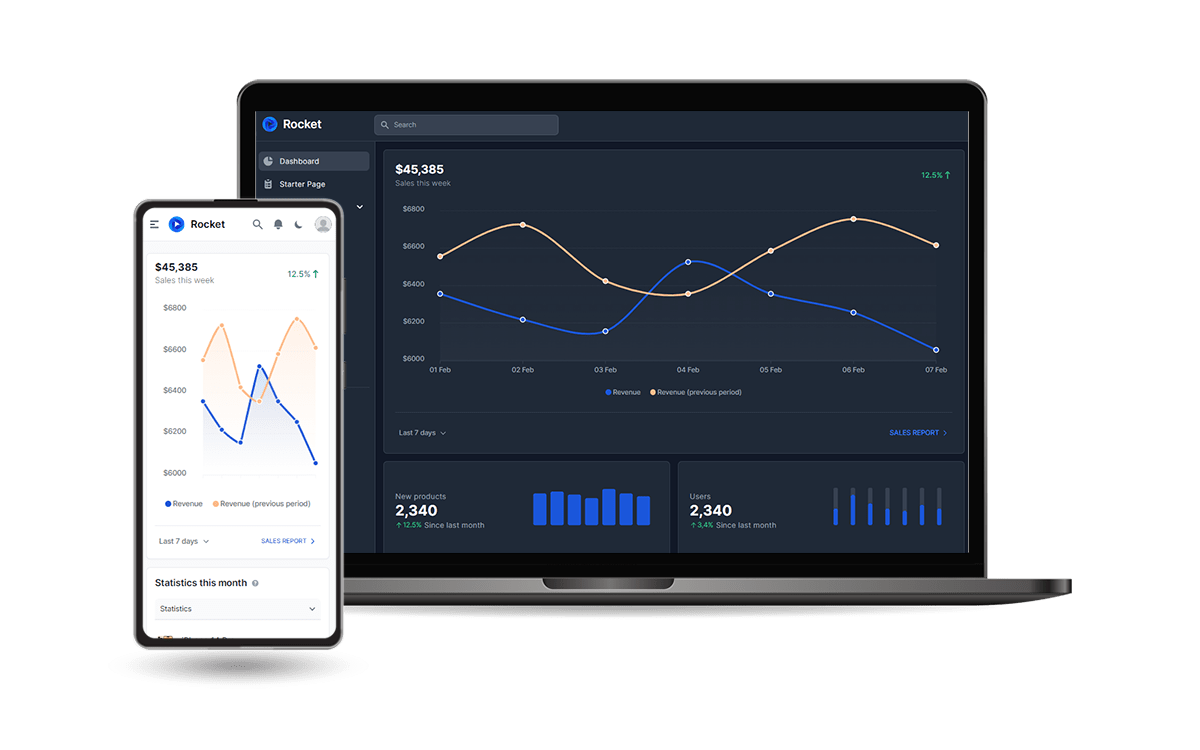
Testing the Hot Reload
Once we download the sources of Rocket Django from the official product page, we need to follow the usual set-up steps for every Django project:
- Open project using VsCode or any other code editor
- Install dependencies
- Migrate database
- Install Tailwind dependencies and watch the CSS files using the command:
$ npx tailwindcss -i ./static/assets/style.css -o ./static/dist/css/output.css --watch
- Start the Django Development server
$ python manage.py runserver
At this point, all changes made on the Python files, templates [ HTML ], and assets [ CSS, JS ] are automatically reflected in the browser:
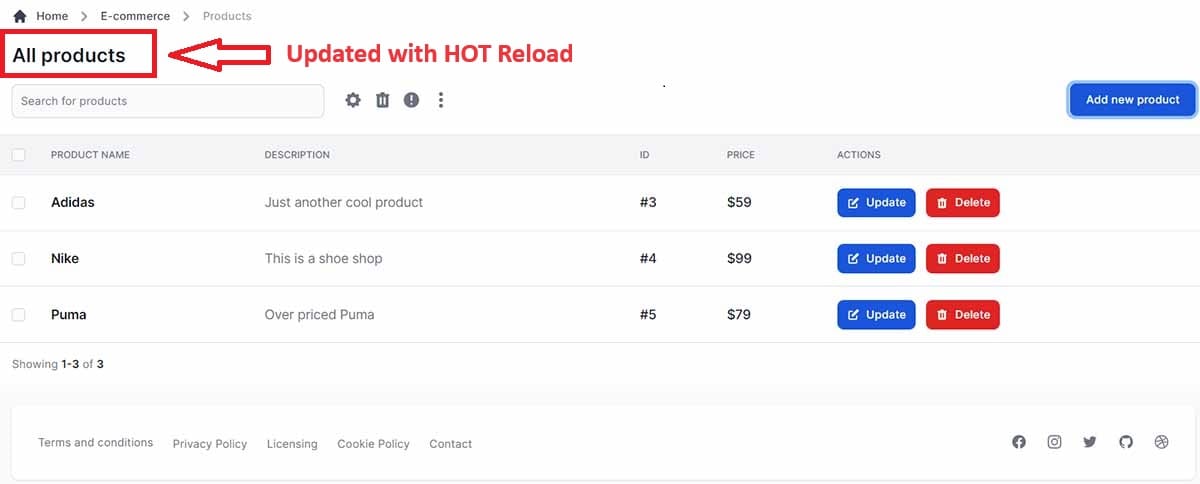
Conclusion
Using the right tooling (hot reload for instance) combined with a good development strategy might help us be more productive and deliver the projects with better quality.
Thanks for reading! For more resources, feel free to access:
- 👉 AppSeed, a platform already used by 8k registered developers
- 👉 Read more about Django Popular Commands (a curated list)
- 👉 Build apps with Django App Generator (free service)